在我们的android中,单选按钮与复选框都是Button的子类,所以继承了Button的各种属性,而且还多了一个可选中的功能。
为什么叫单选按钮呢,因为只能选中一个,所以需要把单选按钮放到我们的按钮组RadioGroup中,从而实现单选功能。
布局代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
| <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/LinearLayout1" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="horizontal" tools:context=".MainActivity" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="请选择性别" android:textSize="23dp" /> <RadioGroup android:id="@+id/radioGroup" android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal"> <RadioButton android:id="@+id/btnMan" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="男" android:checked="true"/> <RadioButton android:id="@+id/btnWoman" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="女"/> </RadioGroup> <Button android:id="@+id/btnpost" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="提交" /> </LinearLayout>
|
运行截图:
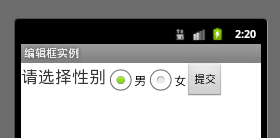
代码解释:
这里的话我们定义了一个TextView,两个radioButton,一个RadioGroup,一个按钮
checked:这个设置单选按钮时否被选中
在RadioGroup中的orientation:"horizontal"
设置按钮组中的按钮按照水平方向进行摆放
接着的话我们通过两种方式获得选中按钮的值
1.单选按钮组的值发生改变时获取,即为RadioGroup设置一个事件监听器:setOnCheckChangeListener
代码:
1 2 3 4 5 6 7 8 9 10 11
| radgroup = (RadioGroup) findViewById(R.id.radioGroup);
radgroup.setOnCheckedChangeListener(new OnCheckedChangeListener() { @Override public void onCheckedChanged(RadioGroup group, int checkedId) { RadioButton radbtn = (RadioButton) findViewById(checkedId); Toast.makeText(getApplicationContext(), "按钮组值发生改变,你选了"+radbtn.getText(), Toast.LENGTH_LONG).show(); } });
|
运行截图:
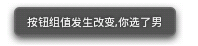
代码分析:
根据按钮组选中值的改变而触发时间,此时onCheckedChanged()方法中的checkedId就是当前选中按钮的id值,直接调用getText方法即可获得对应的值
这里的话要注意一点哦!!!!
按钮组中的单选按钮都要给一个id值哦,不然的话单选功能会失效的
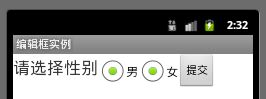
如图,一定要记住哦!!!!
2.单击其他按钮时获取选中的单选按钮的值
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| btnchange= (Button) findViewById(R.id.btnpost); radgroup = (RadioGroup) findViewById(R.id.radioGroup);
btnchange.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { for(int i = 0;i < radgroup.getChildCount();i++) { RadioButton rd = (RadioButton) radgroup.getChildAt(i); if(rd.isChecked()) { Toast.makeText(getApplicationContext(), "点击提交按钮,获取你选择的是:"+rd.getText(), Toast.LENGTH_LONG).show(); break; } } } });
|
运行截图:
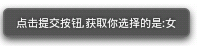
代码解释:
这里我们为提交按钮设置了一个setOnClickListener事件监听器,每次点击的话遍历一次RadioGroup判断哪个按钮呗选中
.getChildCont()
获得按钮组中的单选按钮的数目
.getChinldAt(i)
:根据索引值获取我们的单选按钮
isChecked
:判断按钮是否选中
【另】获取选中的RadioButton控件:
4RadioButton radioButton = (RadioButton)findViewById(radioGroup.getCheckedRadioButtonId());
二.CheckBox复选框
相比于RadioButton,复选框获取值的话就有点麻烦了,因为用户可以多选,所以必须为每个复选框都设置一个setOnCheckedChangeListener
布局代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| <?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="选择你喜欢的颜色" /> <CheckBox android:id="@+id/cboxwhite" android:text="白色" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <CheckBox android:id="@+id/cboxblack" android:text="黑色" android:layout_width="wrap_content" android:layout_height="wrap_content"/> <CheckBox android:id="@+id/cboxgreen" android:text="绿色" android:layout_width="wrap_content" android:layout_height="wrap_content" /> <Button android:id="@+id/btnpost" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="ok" /> </LinearLayout>
|
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63
| private Button btnchange; private CheckBox cboxWhite; private CheckBox cboxBlack; private CheckBox cboxGreen; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnchange = (Button) findViewById(R.id.btnpost); cboxWhite = (CheckBox) findViewById(R.id.cboxwhite); cboxBlack = (CheckBox) findViewById(R.id.cboxblack); cboxGreen = (CheckBox) findViewById(R.id.cboxgreen); cboxBlack.setOnCheckedChangeListener(checkBox_Listener); cboxWhite.setOnCheckedChangeListener(checkBox_Listener); cboxGreen.setOnCheckedChangeListener(checkBox_Listener); btnchange.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { String choose = ""; if(cboxBlack.isChecked()) choose += cboxBlack.getText().toString()+" "; if(cboxWhite.isChecked()) choose += cboxWhite.getText().toString()+" "; if(cboxGreen.isChecked()) choose += cboxGreen.getText().toString()+" "; Toast.makeText(MainActivity.this, choose, Toast.LENGTH_LONG).show(); } }); } private OnCheckedChangeListener checkBox_Listener = new OnCheckedChangeListener() { @Override public void onCheckedChanged(CompoundButton buttonView, boolean isChecked) { CheckBox box = (CheckBox) buttonView; Toast.makeText(getApplicationContext(), "获取的值:" + isChecked + "xxxxx" + box.getText(), Toast.LENGTH_LONG).show(); if(isChecked); } };
|
运行截图:

代码解释:
这里提示一点,就是我们的findViewByID要在布局加载进去之后才使用,不然会报空指针异常,笔者就曾经在此纠结N久
这里的话我们自定义一个OnCheckedChangeListener的对象,然后为每个复选框添加这个对象作为监听器的参数
最后为Btnpost设置一个setOnclickListener,通过ischecked依次判断是否选中,最后使用toast输出选中的信息