Gallery画廊视图:
Gallery和前面学习的Spinner都是一个列表框,他们都继承AbsSpinner,前者显示是一个垂直的列表,后者是一个水平的列表
还有一个重要的区别:spinner是供用户选择,而Gallery的话可以通过拖动来查看列表项
实例:照片查看器
代码:
step 1:先定义我们的main.xml布局文件
定义一个ImageView和Gallery
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <ImageView android:id="@+id/imgshow" android:layout_width="300dp" android:layout_height="300dp" /> <Gallery android:id="@+id/gallery" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="25dp" android:spacing="2pt" android:unselectedAlpha="0.6" />
|
代码解释:
spacing:设置图片之间的间距,这里我们设置gallery每个列表项的距离为2pt
unselectedAlpha:设置当前没被选中的图片的透明度,取值范围是0~255,越小越透明
还有一个属性:android:animationDuration:设置列表项切换时的动画持续时间,单位是毫秒
step 2:
在res\values下新建一个attr.xml的文件,在文件中定义一个stayleable对象,用于组合多个属性
里面指定了一个系统自带的android:galleryItemBackground属性,用于设置个选项的背景
代码:
1 2 3 4 5 6
| <?xml version="1.0" encoding="utf-8"?> <resources> <declare-styleable name = "Gallery"> <attr name = "android:galleryItemBackground"/> </declare-styleable> </resources>
|
step 3:
在MainActivity中创建BaseAdapter对象,重写四个抽象方法,最主要是重写getView的方法
定义一个imageview,设置缩放方式,设置布局,图片,最后返回这个imageView
代码:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81
| package com.jay.demo; import android.os.Bundle; import android.view.View; import android.view.ViewGroup; import android.view.ViewGroup.LayoutParams; import android.widget.AdapterView; import android.widget.AdapterView.OnItemSelectedListener; import android.widget.BaseAdapter; import android.widget.Gallery; import android.widget.ImageView; import android.app.Activity; import android.content.res.TypedArray; public class MainActivity extends Activity { private int[] imgids = new int[] { R.drawable.meinv1,R.drawable.meinv2,R.drawable.meinv3,R.drawable.meinv4, R.drawable.meinv5,R.drawable.meinv6,R.drawable.meinv7,R.drawable.meinv8, R.drawable.meinv9,R.drawable.meinv10, }; private ImageView imgshow; private Gallery gallery; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); imgshow = (ImageView) findViewById(R.id.imgshow); gallery = (Gallery) findViewById(R.id.gallery); BaseAdapter base = new BaseAdapter() { @SuppressWarnings("deprecation") @Override public View getView(int position, View convertView, ViewGroup parent) { ImageView imgView = new ImageView(getApplicationContext()); imgView.setImageResource(imgids[position]); imgView.setScaleType(ImageView.ScaleType.FIT_XY); imgView.setLayoutParams(new Gallery.LayoutParams(75,100)); TypedArray typedArray = obtainStyledAttributes(R.styleable.Gallery); imgView.setBackgroundResource(typedArray.getResourceId(R.styleable.Gallery_android_galleryItemBackground, 0)); return imgView; } @Override public long getItemId(int position) { return position; } @Override public Object getItem(int position) { return position; } @Override public int getCount() { return imgids.length; } }; gallery.setAdapter(base); gallery.setOnItemSelectedListener(new OnItemSelectedListener() { @Override public void onItemSelected(AdapterView<?> parent, View view, int position, long id) { imgshow.setImageResource(imgids[position]); } @Override public void onNothingSelected(AdapterView<?> arg0) { } }); } }
|
运行截图:
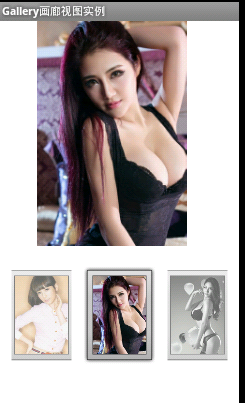
代码中有一些是固定的写法,有个印象即可,以后会深入地说明下…