Laravel 5.2自带用户注册和登录认证,安装好Laravel后,在app根目录执行php artisan make:auth
,即可自动安装一个简易的功能完整的用户认证系统。
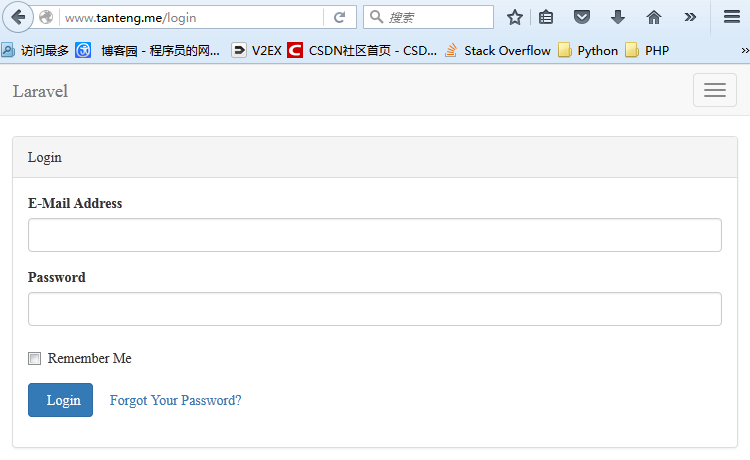
在路由定义文件routes.php中,发现多了一个路由配置:
1 2 3 4 5
| Route::group(['middleware' => 'web'], function () { Route::auth();
Route::get('/home', 'HomeController@index'); });
|
因此输入www.example.com/home
,由于用户没有登录自动转向到登录也没,如上图所示。
看看HomeController控制器:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| <?php namespace App\Http\Controllers; use App\Http\Requests; class HomeController extends Controller { public function __construct() { $this->middleware('auth'); } public function index() { return view('home'); } }
|
很简单,使用auth中间件,如果通过auth中间件则显示home视图。
那么重点就是auth中间件。
在Kernel.php中查看auth中间件的定义:
1 2 3 4 5 6
| protected $routeMiddleware = [ 'auth' => \App\Http\Middleware\Authenticate::class, 'auth.basic' => \Illuminate\Auth\Middleware\AuthenticateWithBasicAuth::class, 'guest' => \App\Http\Middleware\RedirectIfAuthenticated::class, 'throttle' => \Illuminate\Routing\Middleware\ThrottleRequests::class, ];
|
打开app/Http/Middleware/Authenticate.php
文件查看handle方法:
1 2 3 4 5 6 7 8 9 10 11 12
| public function handle($request, Closure $next, $guard = null) { if (Auth::guard($guard)->guest()) { if ($request->ajax()) { return response('Unauthorized.', 401); } else { return redirect()->guest('login'); } } return $next($request); }
|
这里使用了Laravel自带的用户认证Auth,如果没有登录跳转到login,可以login路由在哪定义的呢?还有www.example.com/regisger
等路由没看到定义的地方呀。
在终端执行php artisan route:list
发现注册了这么多路由,包括很多认证的路由:
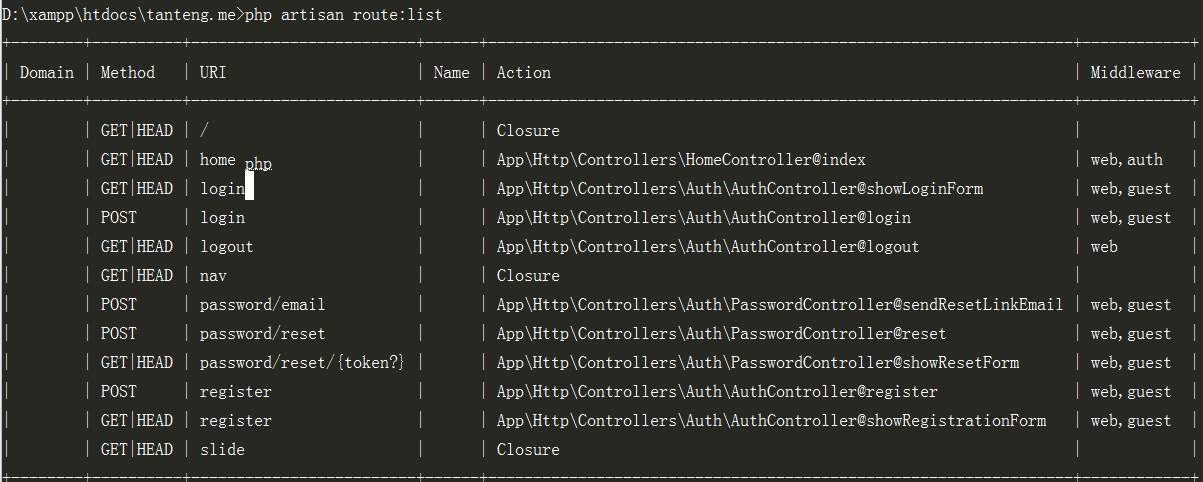
这些路由是经过前面路由文件中的Route::auth()
来进行注册的。
auth方法具体位置vendor/laravel/framework/src/Illuminate/Routing/Router.php
,其中auth方法:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public function auth() { $this->get('login', 'Auth\AuthController@showLoginForm'); $this->post('login', 'Auth\AuthController@login'); $this->get('logout', 'Auth\AuthController@logout'); $this->get('register', 'Auth\AuthController@showRegistrationForm'); $this->post('register', 'Auth\AuthController@register'); $this->get('password/reset/{token?}', 'Auth\PasswordController@showResetForm'); $this->post('password/email', 'Auth\PasswordController@sendResetLinkEmail'); $this->post('password/reset', 'Auth\PasswordController@reset'); }
|
当你登录过再次进入www.example.com/login
,会被自动跳转到首页,这里也是用到了中间件,如上面列出的中间件中的guest,看看app/Http/Middleware/RedirectIfAuthenticated.php
中的handle方法:
1 2 3 4 5 6 7 8
| public function handle($request, Closure $next, $guard = null) { if (Auth::guard($guard)->check()) { return redirect('/'); } return $next($request); }
|
Laravel本身自带了用户注册、登录、密码重置等基本的用户验证功能,并且自带认证方法,视图,路由,开箱即用。